An ongoing problem developing SharePoint custom components is that there is no easy way to help the user with spell-checking. SharePoint does provide some native options for spell-checking, however, this functionality is not provided if you’re making your own WebParts. The Bing Spell Check is an Azure Service that can be easily implemented, and it’s fast and cheap. In this article, we will examine some background information about the service and basic implementation in SharePoint, and where there’s room for further refinement.
The Bing Spell Check
One of the Cognitive services in Azure is the Bing Spell Check. The service exposes two APIs, one as REST and the other as a Microsoft SDK. Bing Spell Check detects and analyzes the text which has been inputted by the user, it then indicates whether there is a spelling error and makes recommendations to improve the grammar or orthography.
The traditional approach for spell-checking, like the methods used in Microsoft Word, is based on dictionaries. There are several lists with words and dictionary-based rule sets, and each word in the text is verified against them. This is a static method that doesn’t consider different contexts, therefore meaning the user and editorial staff must maintain the dictionary.
The latest version of the Bing Spell Check (currently in the 3rd generation) is an AI dynamic system that, according to Microsoft, “…leverages machine learning and statistical machine translation to dynamically train a constantly evolving and highly contextual algorithm”. Bing Spell Check takes into consideration not only the writing errors but also the context of words in the text by recognizing slang and popular expressions. The service now supports 40 languages and regions and has two pricing tiers. A free tier which includes 1 call per second and 1000 calls per month, and a paid tier which includes 100 calls per seconds and charges for every 10,000 calls.
There are also two Modes for the service: Proof provides the most comprehensive checks, capitalization, and basic punctuation, but is only available for English, Spanish and Portuguese. The Spell mode, available for all supported languages, finds most of the spelling mistakes, but not all of the grammatical errors.
Creation of the service in Azure
From the Azure administrators’ panel, log in with your administrator credentials, add or select a Resource Group and add the Bing Spell Check v7 service. Next, open the properties panel, go to Keys and copy the KEY 1 value.
Integration in SharePoint
To demonstrate this functionality, we can create a SharePoint Framework (
In the next example, the SPFx WebPart shows a simple interface, giving text boxes to define the text to check, the Market (language and dialect) and Mode. Using a button, the corrected text, if any, is shown in a label. In the example, the REST API is used and, although the SPFx WebPart can make a REST-call directly to Azure, we are making a call to an Azure Function that makes the final call to the service; this allows us to use CSharp and make a more general example of use. The
The Run method in the Function extracts the input parameter values and calls a CheckSpelling routine, that has the following form:
static async Task<AzureSpellCheckResults> CheckSpelling(
string StringToCheck, string Market, string Mode)
{
string servEntryPoint = "https://api.cognitive.microsoft.com/bing/v7.0/spellcheck?";
string servKey = "1c7b01f6f5dc...400";
HttpClient servClient = new HttpClient();
servClient.DefaultRequestHeaders.Add("Ocp-Apim-Subscription-Key", servKey);
HttpResponseMessage servResponse = new HttpResponseMessage();
string servUri = servEntryPoint + "mkt=" + Market + "&mode=" + Mode;
List<KeyValuePair<string, string>> servInput = new List<KeyValuePair<string, string>>();
servInput.Add(new KeyValuePair<string, string>("text", StringToCheck));
using (FormUrlEncodedContent servContent = new FormUrlEncodedContent(servInput))
{
servContent.Headers.ContentType = new MediaTypeHeaderValue("application/x-www-form-urlencoded");
servResponse = await servClient.PostAsync(servUri, servContent);
}
string servResult = await servResponse.Content.ReadAsStringAsync();
return JsonConvert.DeserializeObject<AzureSpellCheckResults>(servResult);
}
Here
The servEntryPoint is the base URL for the Azure Bing Spell Check REST API, and it is a fixed value:(“https://api.cognitive.microsoft.com/bing/v7.0/spellcheck”). Set the Key value, found earlier in the Service-instance, in the variable
public class AzureSpellCheckResults
{
public string _type { get; set; }
public Flaggedtoken[] flaggedTokens { get; set; }
}
public class Flaggedtoken
{
public int offset { get; set; }
public string token { get; set; }
public string type { get; set; }
public Suggestion[] suggestions { get; set; }
}
public class Suggestion
{
public string suggestion { get; set; }
public float score { get; set; }
}
The text is examined by the Bing service word by word. If one word is considered as an error, it gets a Flaggedtoken and a collection of Suggestions. Each suggestion consists of a corrected word and a Score, from 0 to 1, that indicates how successful the service considers the suggestion (1 is a full match).
In the example is an auxiliary routine ModifyText used to replace the errors with the first recommendation (always the suggestion with the highest score):
static string ModifyText(string InputString, AzureSpellCheckResults SpellingResult)
{
foreach (Flaggedtoken oneWord in SpellingResult.flaggedTokens)
{
InputString = InputString.Replace(oneWord.token, oneWord.suggestions[0].suggestion);
}
return InputString;
This is a very basic function, but it works for demonstrating the concept. In a more elaborate implementation, the interface would show the words with errors and allow the user to select from different suggestions.
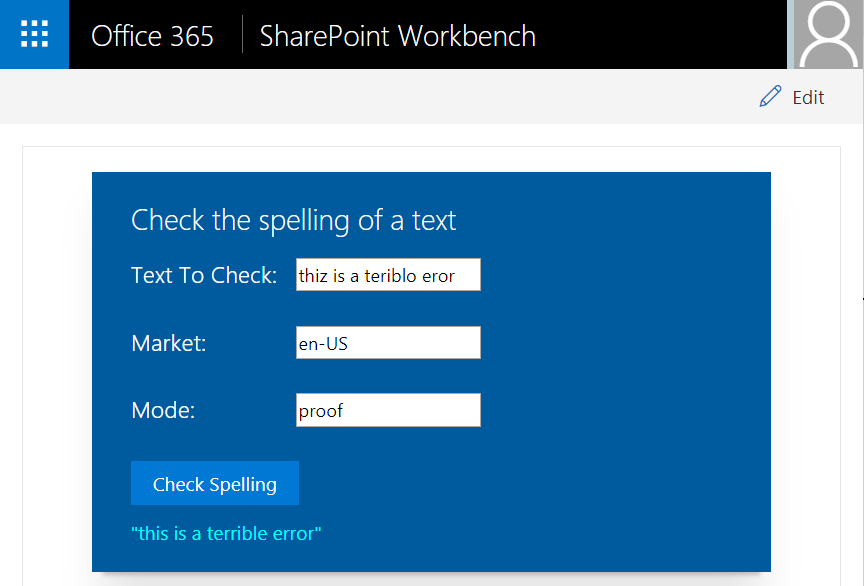
In summary, the Azure Bing Spell Check offers an inexpensive and easy-to-use technique, allowing us to integrate orthographical and grammatical spelling control in SharePoint customizations. Additionally, the AI algorithms in the service ensure that the quality of the results can only improve in the future and that soon, we won’t need to care about the maintenance of the spelling dictionaries.t
Good day, I would like to speak with someone about integrating Bing Spell check. May I have their contact? My contact is: harriott.myers@fimifont.com. Thanks,