And Tell People That Send Emails to Teams to Use a Different Approach
The nature of Microsoft 365 is that you’re on a voyage of constant discovery. Take the fact that group mailboxes support auto-reply messages, which completely passed me by. Exchange Online has long supported auto-reply for user and shared mailboxes, but not for group mailboxes. Microsoft updated Groups recently to support this capability. At least, I think it was recently, but can’t be sure exactly when.
Having Exchange Online generate an auto-reply is useful when the need exists to inform people about some special processing for messages that arrive in a group mailbox. For example, to:
- Describe the procedure to process messages sent to a group to a sender. For example, a team of agents uses a group mailbox and Outlook to process inbound messages from customers instead of a shared mailbox (the usual approach).
- Tell internal people that a group is team-enabled, and all conversations occur in the team. Team-enabled groups are often hidden from Exchange clients and not listed in the GAL and other address lists, but that doesn’t stop people from sending messages to their SMTP addresses.
- Inform people when a group (or team) is not in active use or archived.
Setting an Auto-Reply for a Group Mailbox
Apart from the inconvenience that Outlook doesn’t support setting an auto-reply for a group mailbox, creating an auto-reply for a group mailbox with PowerShell is just like working with a user mailbox. Exchange Online stores the auto-reply text as HTML and you can include many basic HTML commands when formatting an auto-reply message.
In this example, we use the Set-MailboxAutoReplyConfiguration cmdlet to create an auto-reply for a group mailbox. The message sent to external people tells them that the mailbox is not in use. Because the group is team-enabled, the message used for internal people includes a mailto: link to the email address for a channel in the team. The recipient can click on the link to create and send their message to Teams.
Set-MailboxAutoReplyConfiguration -Identity "Office 365 for IT Pros" -ExternalMessage "Sorry, this mailbox doesn't accept email" -AutoReplyState Enabled -InternalMessage 'Please! We use Teams for communication, so send your message to <a href = "mailto: 943a5092.office365itpros.com@na.teams.ms">Teams</a> and it will be dealt with there.' -ExternalAudience All
Figure 1 shows an auto-reply message received after sending a message to a group mailbox. You can see a link to Teams. This is the mailto: link. Clicking the click causes Outlook to create a new message addressed to the selected channel in the team owned by the group.
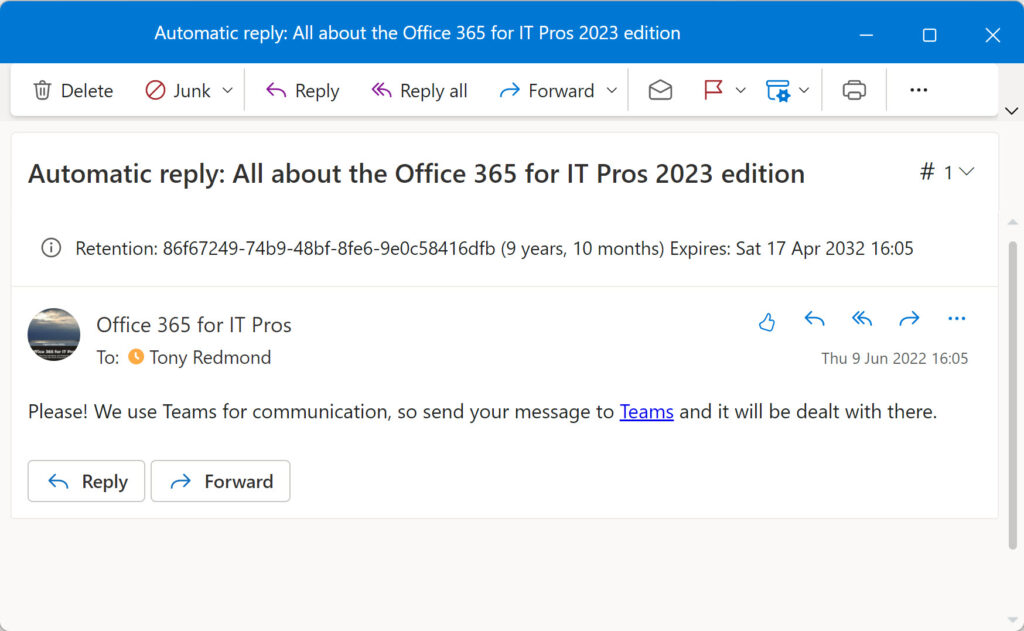
Setting Auto-Reply for All Team-enabled Groups
Creating an auto-reply for a single team-enabled group is easy. Trying to do the same for hundreds of team-enabled groups might become boring, so some automation is necessary. The steps to do the job with PowerShell are straightforward:
- Find the set of team-enabled Microsoft 365 Groups.
- For each group, set an auto-reply.
What’s complicated is knowing what channel in a team to use for email. By default, Teams does not assign email addresses to channels. Any team member can request Teams to provision an email address (and bizarrely, any team member can remove the address). The email address for a channel is separate from the group’s SMTP address and routes messages via special mailboxes. Connectors pick the emails up from the special messages to ingest them into the target channel.
The first difficulty is to find what channels in a team are email-enabled. The second is to decide where to route messages. To make things simple, I decided to look for teams where the General channel is email-enabled. If a team meets this criterion, I assign an auto-reply with the email address of the General channel in the mailto: link. If not, I use some generic text.
Finding Teams
The first step is to find the set of team-enabled groups. You can do this with standard Exchange Online PowerShell by running the Get-UnifiedGroup cmdlet. For example:
[array]$Teams = Get-UnifiedGroup -Filter {ResourceProvisioningOptions -like "*team*"}
However, the Get-UnifiedGroup cmdlet is quite slow, and we can get more speed by using the groups cmdlets from the Microsoft Graph PowerShell SDK. This factor becomes increasingly important when the number of groups in a tenant increases past a few hundred. Using the Get-MgGroup cmdlet, we can fetch the set of team-enabled groups with a command using a lambda filter that looks much like the filter used with the Get-UnifiedGroup cmdlet:
[array]$Teams Get-MgGroup -All -Filter "resourceProvisioningOptions/Any(x:x eq 'Team')"
A nice thing about using Get-MgGroup with the All parameter is that the cmdlet takes care of pagination to retrieve all available groups.
Finding Email-Enabled Team Channels
Next, we loop through each team-enabled group to retrieve the set of channels that have email addresses. The connection to the Graph must have the Group.Read.All permission to read group information and the ChannelSettings.Read.All permission to read channel information. Here’s the code I used.
$Report = [System.Collections.Generic.List[Object]]::new() ForEach ($Team in $Teams) { Write-Host "Processing" $Team.DisplayName $Uri = "https://graph.microsoft.com/v1.0/teams/$($team.id)/channels" [array]$Channels = Invoke-MgGraphRequest -Uri $Uri -Method Get ForEach ($Channel in $Channels.Value) { If (!([string]::IsNullOrWhiteSpace($Channel.Email))) { # We have a channel email address, so report it $ReportLine = [PSCustomObject]@{ Team = $Team.DisplayName Channel = $Channel.DisplayName Email = $Channel.Email TeamId = $Team.Id } $Report.Add($ReportLine) } } # End for each channel } # End for each team
Two points are worth making about the code. First, the Get-MgTeamChannel cmdlet is available to list the channels for a team, but I discovered that the cmdlet returns the group’s primary SMTP address for the General channel instead of the team channel email address. That’s why I use the Invoke-MgGraphRequest cmdlet to run a Graph query using the channels API.
Second, I process all email-enabled channels rather than just the General channel because it can be useful to have a report of all email-enabled channels and their addresses. When the loop completes, I filter the output to generate a list of just the General channels with email addresses:
# Create another array with just email addresses for General channels [array]$GeneralChannels = $Report | Where-Object {$_.Channel -eq "General"}
Setting Auto-Reply
All that’s left is to run down through the set of team-enabled groups to set an appropriate auto-reply. This code checks the array containing the set of email-enabled General channels to retrieve an email address if available. We then have a simple If/Else structure to set the auto-reply with code similar to that explained above.
ForEach ($Team in $Teams) { $EmailAddress = $Null $EmailAddress = $GeneralChannels | Where-Object {$_.TeamId -eq $Team.Id}| Select-Object -ExpandProperty Email If ($EmailAddress) { Write-Host "Setting auto reply with mailto: for" $Team.DisplayName $InternalMessage = 'Please! We use Teams for communication, so send your message to <a href = "mailto:' + $EmailAddress + '">Teams</a> and it will be dealt with there.' Set-MailboxAutoReplyConfiguration -Identity $Team.Id -ExternalMessage "Sorry, this mailbox doesn't accept email" -AutoReplyState Enabled -InternalMessage $InternalMessage } Else { Write-Host "Using default auto-reply for" $Team.DisplayName Set-MailboxAutoReplyConfiguration -Identity $Team.Id -ExternalMessage "Sorry, this mailbox doesn't accept email" -AutoReplyState Enabled -InternalMessage "Please use Teams to communicate with us" -ExternalAudience All } } # End For each team
You can download the full script from GitHub.
Yammer Communities and Auto-Replies
If you use Yammer and the network is configured in Microsoft 365 mode so that communities are based on Microsoft 365 groups, you could take the same approach of finding the groups used by Yammer with a command like:
[array]$YammerGroups = Get-UnifiedGroup -Filter {ResourceBehaviorOptions -like "*yammer*"}
After finding the set of groups, you can update their auto-reply messages appropriately. However, Yammer accepts messages sent by email and posts them to the target communities, so there’s no need to include a mailto: link.
Nice to Have but Not Essential
Setting an auto-reply on a group mailbox is not a necessity. It won’t make a tenant run more smoothly or make Teams work better. It’s just a nice way to communicate with people when the need arises.
I followed your instructions to enable external auto replies from a group and was not able to get it to work.
I ran command Get-MailboxAutoReplyConfiguration and noticed a parameter I didn’t see mentioned in this article:
-ExternalAudience None
I did a little more reading and found this syntax article: https://learn.microsoft.com/en-us/powershell/module/exchange/set-mailboxautoreplyconfiguration?redirectedfrom=MSDN&view=exchange-ps#-externalaudience
Which indicates the default is to not reply to anyone outside of the organization. Changing that parameter to “All” allowed things to work as expected.
The Real Person!
Author Tony Redmond acts as a real person and passed all tests against spambots. Anti-Spam by CleanTalk.
According to the documentation
All: Automatic Replies are sent to all external senders. This is the default value.
I don’t see that the default value is None, meaning don’t send any external replies. But it doesn’t do any harm to be specific.
Hi Tony,
I see what you mean. Though, if you look at little bit below where you quoted, in the table, it says:
“Default value: None”
So their documentation seems to contradict itself.
I personally found the setting on mine to be “None” by default.
It might be one of those things they changed recently and haven’t completely updated their documentation to reflect the change.
The Real Person!
Author Tony Redmond acts as a real person and passed all tests against spambots. Anti-Spam by CleanTalk.
All too true.
Among all the searching for groups vs shared mailboxes you are the best source by far. Thank you!
The Real Person!
Author Tony Redmond acts as a real person and passed all tests against spambots. Anti-Spam by CleanTalk.
Thanks. We do our best to publish practical, usable material on P365.
Awesome! Thank you Tony!
Is there existing PowerAutomate templates or MS Graph API examples where end-users could fill the group (shared) mailboxes’ autoreplies? Looking for “end-user friendly way” to fill such autoreplies as many are not familiar with powershell related scripts.
The Real Person!
Author Tony Redmond acts as a real person and passed all tests against spambots. Anti-Spam by CleanTalk.
Check out https://docs.microsoft.com/en-us/graph/api/resources/automaticrepliessetting?view=graph-rest-1.0
I haven’t used this API, but it looks like the right one.
Is the same possible for Shared Mailboxes?
The Real Person!
Author Tony Redmond acts as a real person and passed all tests against spambots. Anti-Spam by CleanTalk.
Yes.