Every time we do an Intune deployment, especially for clients who come from SCCM or other platforms, we are asked to provide some means to examine details of managed devices. As we all know, Intune reporting is a bit “basic,” so in this article, I walk through the steps to use PowerShell to obtain the information commonly requested by clients to support their operations.
Intune PowerShell Modules
Currently, two PowerShell modules can be used. The first is the Intune PowerShell module; the second is the Microsoft Graph PowerShell SDK, which includes the device management and applications sub-modules. Microsoft doesn’t maintain the Intune PowerShell module anymore and I recommend that you use the Microsoft Graph PowerShell SDK one, which Microsoft actively maintains to aligned with the latest release of Graph APIs.
The scripts we develop using the Graph SDK are more future-proofed and it will be easier to add new features along the road. Another benefit from using the Graph SDK is that it works for non-Windows devices, which is handy for people like me who use macOS devices as their primary workstation.
To start, we must connect the Graph API endpoint using Connect-MgGraph cmdlet. In case you need it, here are the steps to install the Microsoft Graph PowerShell module, and some advice about different means to authenticate and connect to the Microsoft Graph PowerShell modules is available found here. Note that we just need to install Microsoft.Graph PowerShell modules as the rest will be loaded on-demand when needed.
Connect-MgGraph -Scopes "DeviceManagementApps.Read.All","DeviceManagementManagedDevices.Read.All"
You may now wonder what scopes (permissions) are needed to run the device management cmdlets. I recommend that you first identify the cmdlet you want to use then check the permission scope required from the Microsoft documentation. For what we need to do, the following scopes should be enough.
- DeviceManagementApps.Read.All
- DeviceManagementManagedDevices.Read.All
Now we are ready to gather data about devices. I will separate the sections into the different types of reports we usually generate.
Remember to use PowerShell 7 or above to run Microsoft Graph PowerShell SDK cmdlets. Using Windows PowerShell may result in the following error, which has yet to be fixed by Microsoft (Figure 1).
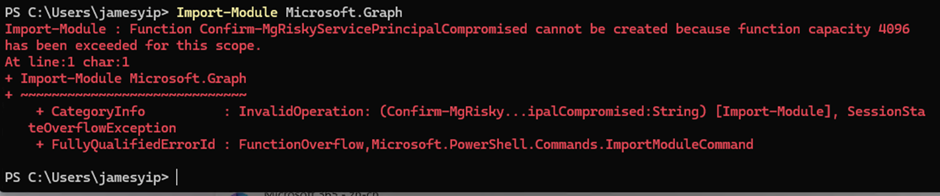
Get List of Applications for Managed Devices
I have different reports for mobile and Windows devices as the data available differs across device families. For mobile devices, two management approaches are available: Mobile Application Management (MAM) and Mobile Device Management (MDM) with different device management capabilities. I don’t discuss the differences here, but you can refer to this article for more details.
Clients often ask for a report about managed applications and versions and expect that the report includes both MAM and MDM managed applications . The Graph SDK cmdlets you need are use are:
- Get-MgDeviceManagementDetectedApp (MDM application data covering Windows, macOS, iOS, and Android)
- Get-MgDeviceAppManagementManagedAppRegistration (MAM).
Here’s some example code that I use to pull a report of all apps from mobile devices managed by Intune MAM (including Azure AD joint device):
$result=@ () Get-MgDeviceManagementDetectedApp -All | ForEach-Object { $tmp=$_ $result+=(Get-MgDeviceManagementDetectedAppManagedDevice -DetectedAppId $_.id | Select-Object -Property @{Name=”Device”;Expression={$_.DeviceName}}, @{Name=”App”;Expression={$tmp.DisplayName}}, @{Name=”Version”;Expression={$tmp.Version}}, @{Name=”Platform”;Expression={$tmp.platform}}) } $result | Sort-Object -Property Device, App, Version | Out-GridView
The result looks like the output shown in Figure 2.
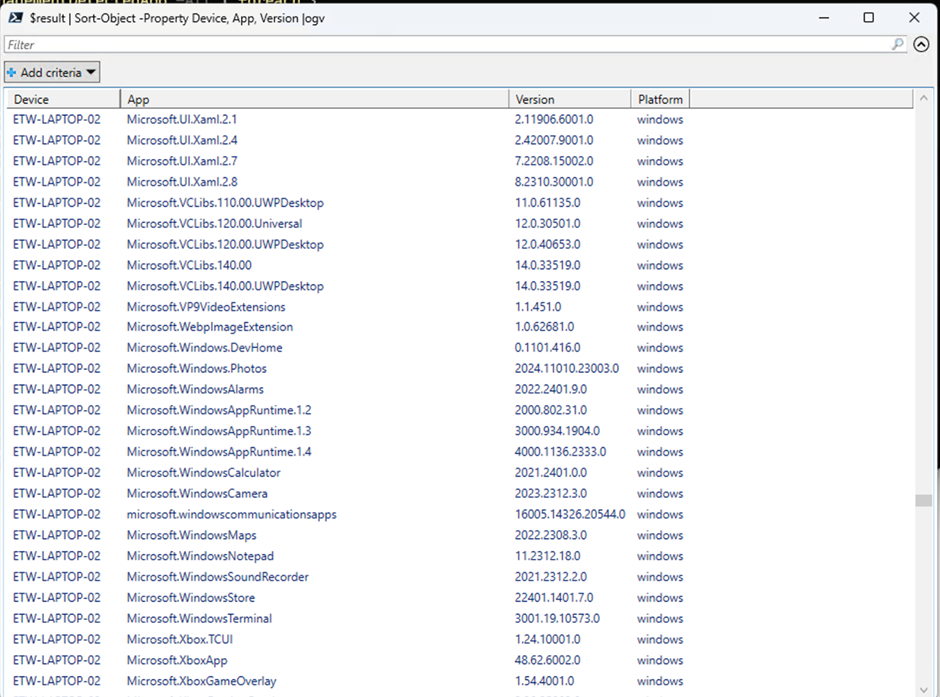
Let me explain the script flow:
- We define an empty array called tmp to store the result.
- Use Get-MgDeviceManagementDetectedApp -All to get all the detected app from Intune.
- The ForEach-Object cmdlet processes the app data and calls Get-MgDeviceManagementDetectedAppManagedDevice using the ID for each app record we obtained from the previous step.
- Inside the foreach loop, the script selects the required properties from the result and constructs a custom object.
- The script then uses Sort-Object to sort the output according to your needs.
- Finally, the script displays the data using Out-GridView. You can also use another cmdlet like Export-Csv to output to a CSV file.
Get List of Applications for Managed Apps
We can use Get-MgDeviceAppManagementManagedAppRegistration to fetch MAM registration information (figure 3). Registration information means the apps registered in Intune MAM.
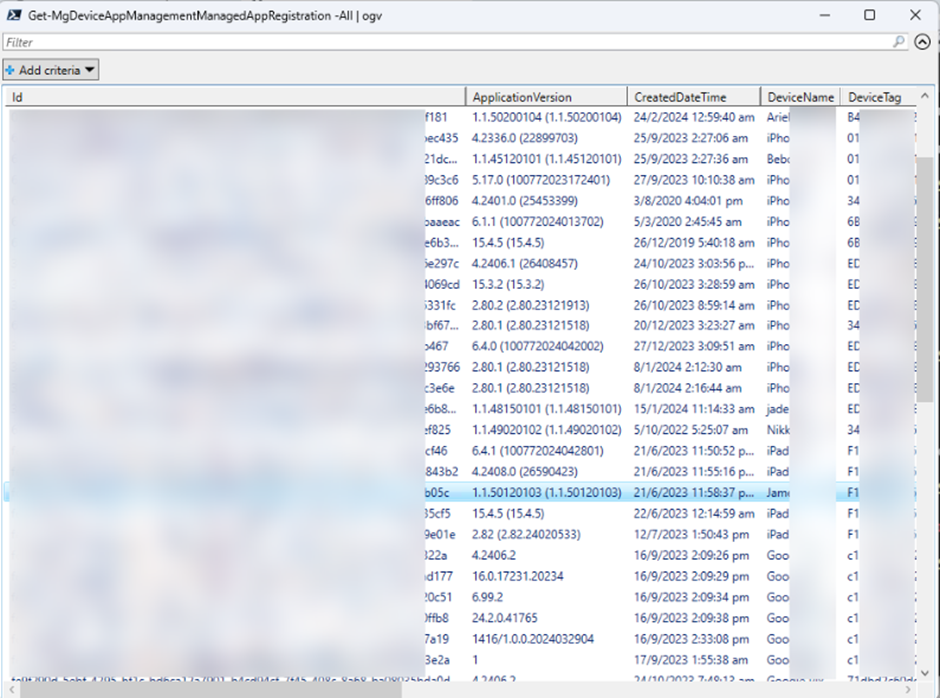
However, some data that this cmdlet returns may not be in a meaningful format, as illustrated in Figure 4. Result data does not include the application name and outputs an object type name. You may need to add logic to handle this kind of situations to obtain the underlying data. For example, try to use Select-Object -ExpandProperty to expand the data or create custom objects as part of your PowerShell script.
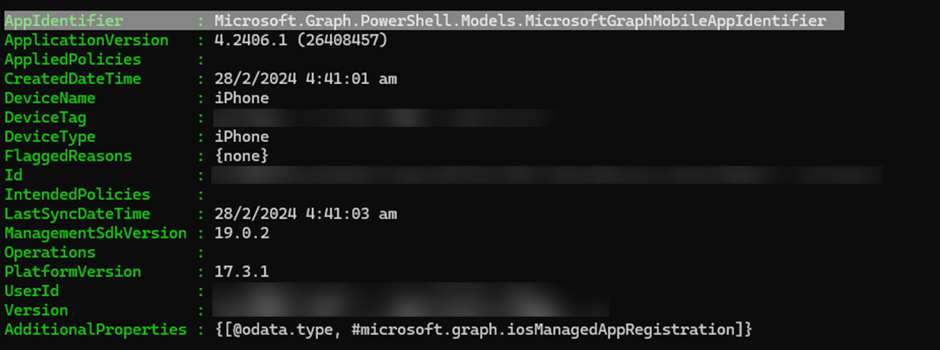
According to the Microsoft’s documentation, the app identifier should show the application’s ID, like com.microsoft.office.outlook.ios. But it’s not showing needed info, instead it is showing object type names like Microsoft.Graph.PowerShell.Models.MicrosoftGraphMobileAppIdentifier, and this makes us unable to make good use of the data returned.
Further Processing the Data
PowerShell includes some handy ways to combine data. I always like to use the Join-Object cmdlet to combine data sets to enhance the report. For example, Get-MgDeviceAppManagementManagedAppRegistration returns UserId which means nothing to our users. We can then use Get-MgUser to get a list of licensed users and combine using UserId. I usually use Join-Object to do SQL-like JOIN operations, as it’s using LINQ behind the scenes so it performs much faster when a dataset is large. Note that Join-Object is not a built-in cmdlet, you need to use Install-Module Join-Object to install it first. Details about the Join-Object cmdlet are available here. After that, you can use script like below to combine data:
$users=Get-MgUser -All -Filter "assignedLicenses/`$count ne 0 and userType eq 'Member'" $mam=Get-MgDeviceAppManagementManagedAppRegistration -All Join-Object -Left $mam -Right $users -LeftJoinProperty "UserId" -RightJoinProperty "Id" -ExcludeLeftProperties "CreatedDateTime","AdditionalProperties" -RightProperties "DisplayName" | Out-GridView
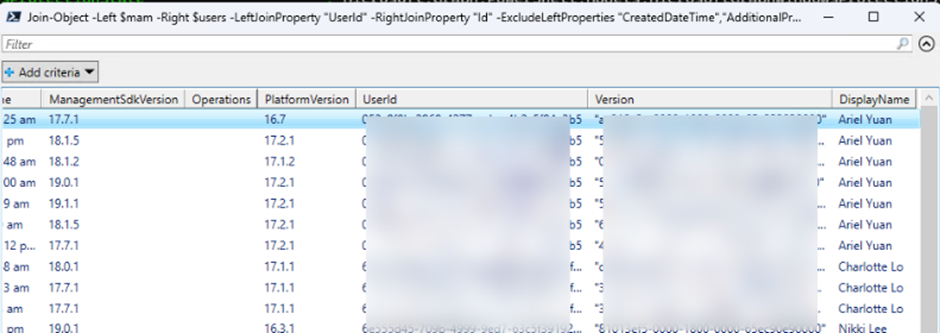
The script above performs the following:
- Gets the licensed user list from Get-MgUsers and stores in variable.
- Gets the Intune MAM registration information using Get-MgDeviceAppManagementManagedAppRegistration and stores in variable.
- Uses Join-Object cmdlet to do a SQL-like join with UserId properties and only includes needed field Display Namse from data collected in step 1.
- Displays the result in Out-GridView
Unlimited Possibilities for Graph Reporting
With the help of Join-Object cmdlet, you can easily combine data retrieved using different PowerShell cmdlets instead of exporting data to CSV and using Excel to do the massaging. But note that processing like Join-Object happens in memory, so make sure you have enough free memory. You can check the free memory information by looking at Task Manager and see how much memory is consumed by pwsh.exe and how much free memory is available in your system.
TEC Talk: Boost your M365 Administration & Security with AI
Join Curtis Johnston and Habib Mankal’s Free Webinar on March 19th @ 11 AM EST.