Introducing GitHub Copilot
Microsoft is standardizing the Microsoft Graph API for programmatic access to Microsoft 365 tenant configuration, applications, and user data instead of relying on older PowerShell modules like Azure AD, Azure AD Preview, and MSOL. In fact, these three modules are being deprecated. While the deprecation date has moved, organizations should plan to update their scripts so that they no longer depend on these modules.
GitHub Copilot can help with the upgrade. GitHub Copilot is an AI-powered code completion and suggestion tool developed by GitHub in collaboration with OpenAI, the makers of ChatGPT. It assists anyone writing code by providing real-time code suggestions and auto-completion based on natural language comments and context within code editors. The combination of code suggestions and Copilot’s understanding of PowerShell can help tenant administrators convert their legacy scripts to use cmdlets from the Microsoft Graph PowerShell SDK. It is important to note that GitHub Copilot is only a tool. It cannot replace fundamental understanding of how the Microsoft Graph works. For those interested in understanding the Microsoft Graph SDK, Practical 365 has an extensive collection of articles covering the use of the Microsoft Graph PowerShell SDK.
This Quickstart for Github Copilot guides you through signing up and installing the plug-in for Visual Studio Code. If you haven’t already signed up, there is a free 30-day trial. After the 30 days, the fee is $10.00/month. There is a lot that you can do in 30 days, so it’s worth testing GitHub Copilot.
Before You Begin to Update a Script
There are two things that should be done before touching any code in a script:
- Understand what the script does.
- Test what the script does.
Maybe the script was put into production before you joined the organization. Maybe the script author recently left the organization. If you don’t know what the script does, and you make changes, how can you know if it still does what it is supposed to do? Did the script throw an error every 10th time it ran before updating the code or is this a new problem?
A suite of tests could act as a simple checklist to make sure the script does everything it is supposed to do. Depending on the script, it might be prudent to check and make sure it’s not doing anything it shouldn’t be doing. If you are interested in automating tests, consider using Pester. Whether the tests are automated or not, you must know what the expected outcomes are so that you can compare them to the results of your updated scripts.
The bottom line is that once you begin to update code, you must know what works and what doesn’t before making any changes.
Updating Script Code with GitHub Copilot
To update a script, do not throw all the code at CoPilot and say “Rewrite this using the Microsoft Graph PowerShell SDK.” Instead, review the code and figure out where cmdlets from legacy modules are used. From there, use Copilot to replace those lines of code.
I have a script similar to “How to Create a Microsoft 365 Licensing Report using the Microsoft Graph SDK for PowerShell” that generates a license inventory report for a Microsoft 365 tenant. The script uses the MSOL module, connects to Microsoft 365 using the Connect-MSOLService cmdlet, gets the product SKUs, and generates a report based on the number of available licenses. This script is approximately 350 lines of code. However, the core of what needed to change was only about 10 lines of code. I copied those lines of code into a temporary script file and started interacting with Copilot.
The code below is an extract of some of the original code. Rather than generating a full HTML report, this snippet writes out the variables so I can test the output of the old version and compare it to the new version.
Connect-MSOlService $licenses = Get-MSOlAccountSku Foreach ($license in $licenses) { if($license.AccountSkuId -eq "andylanddev:SPE_E3") { $spe3Active = $license.ActiveUnits $spe3Warning = $license.WarningUnits $spe3Consumed = $license.ConsumedUnits } if($license.AccountSkuId -eq "andylanddev:SPE_E5") { $spe5Active = $license.ActiveUnits $spe5Warning = $license.WarningUnits $spe5Consumed = $license.ConsumedUnits } } $spe3Warning $spe3Active $spe3Consumed $spe5Warning $spe5Active $spe5Consumed
After completing the Quickstart for Github Copilot, you should have the Copilot Chat Visual Studio Code extension installed.
In Figure 1, I selected the code shown above and prompted Copilot Chat with “Update this code so that it uses the Microsoft.Graph PowerShell Module rather than the MSOL Module.”
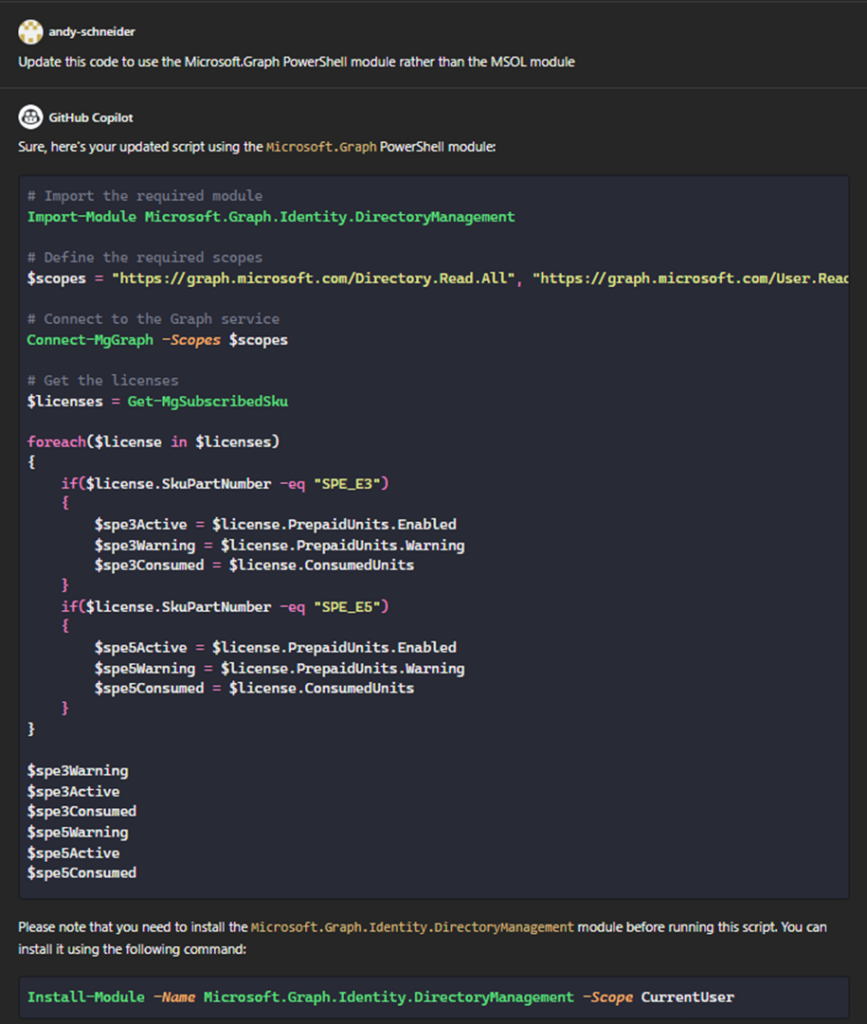
Copilot performed several actions to upgrade the code. First, it replaced the Get-MSOLAccountSku cmdlet with Get-MgSubscribedSku. Copilot also knew that the property AccountSkuId must be replaced with SkuPartNumber.
I copied the new code below the old code in demoscript.ps1 to make it easy to compare the output (Figure 2)
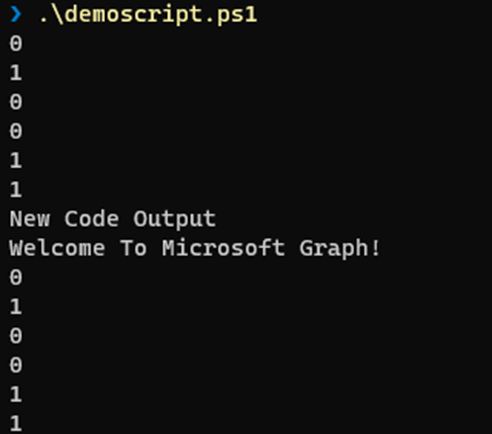
It’s not obvious from this screenshot, but one issue that I ran into was the scope that Copilot used was not correct. Copilot suggested that Directory.Read.All be used. However, the documentation for the List subscribed SKUs API notes that this API call requires the Organization.Read.All permission. In addition to the official Microsoft documentation, read this article on How to Figure Out What Microsoft Graph Permissions You Need, for a detailed explanation on determining scopes required for Cmdlets.
I find myself “chatting” with GitHub Copilot almost as if it is a pair programming partner. I wanted to make sure that the next time I asked, it would provide the correct scope.
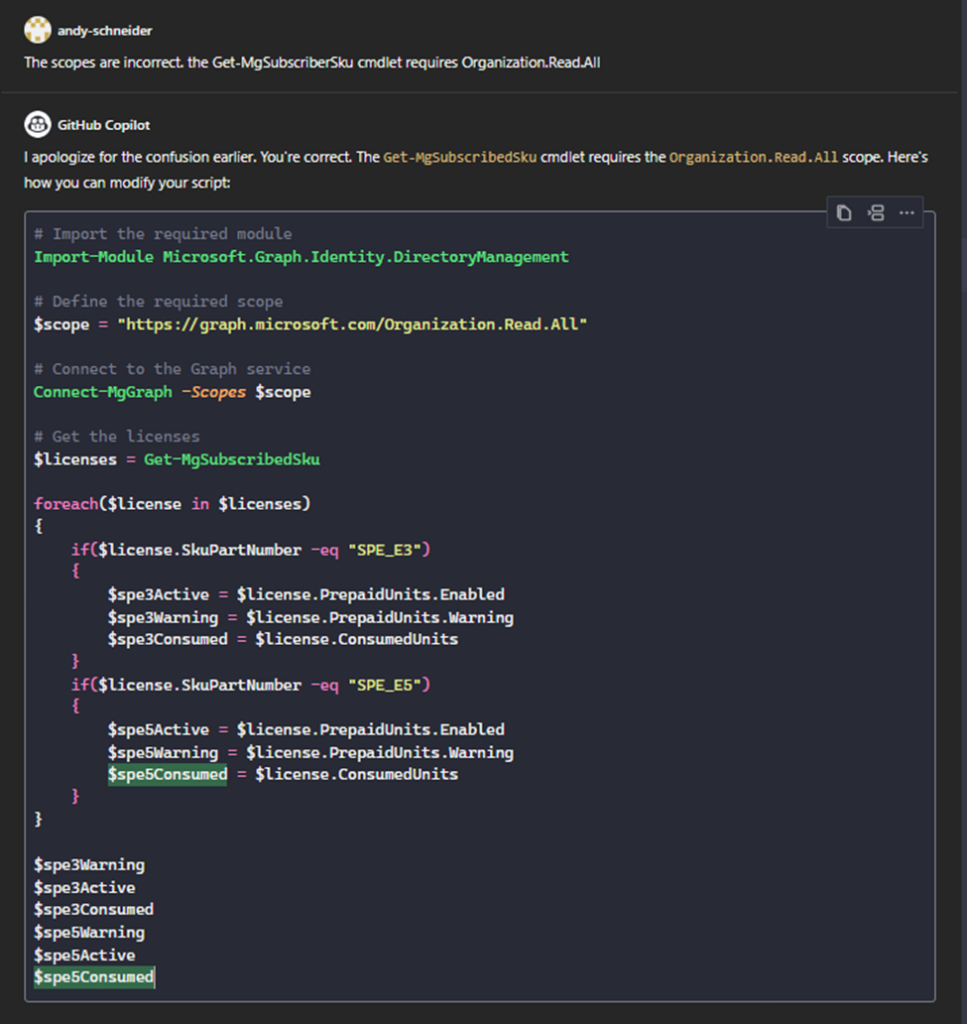
Although I had the right scopes, Github Copilot wrote out the full URI of the scope, shown in Figure 2. I changed this to use the shorter version, to Organization.Read.All (Figure 3). I also added the “NoWelcome” parameter switch to minimize output when the script connects to the graph. Other than that, the output of the old and new scripts was the same. When you are comfortable with the tests passing, you can start testing the entire script to upgrade it to the Microsoft Graph PowerShell SDK.
A copy of the original code and the updated version can be found on this Github Gist.
Github Copilot Improves Steadily
When I started using GitHub Copilot a little over a year ago, I gave up on it after about a week of playing. It was a cute toy, but it caused more problems than it solved. About 6 months ago, I decided to give it another try and the code completion improvements were incredible. The suggestions were spot on about 90% of the time.
However, the feature that finally got me to start paying $10/month is GitHub Copilot Chat, which was announced at Microsoft Build earlier this year and it is now available to everyone. Check out this article for details about the announcement. With the introduction of Copilot Chat, I no longer need to memorize a bunch of keyboard shortcuts or go digging through menus in my IDE to refactor code. I can simply ask Copilot Chat to “Add comment-based help to this function” and it adds the help automatically and provides a great starting point for a description and synopsis of the function I am writing (Figure 4).
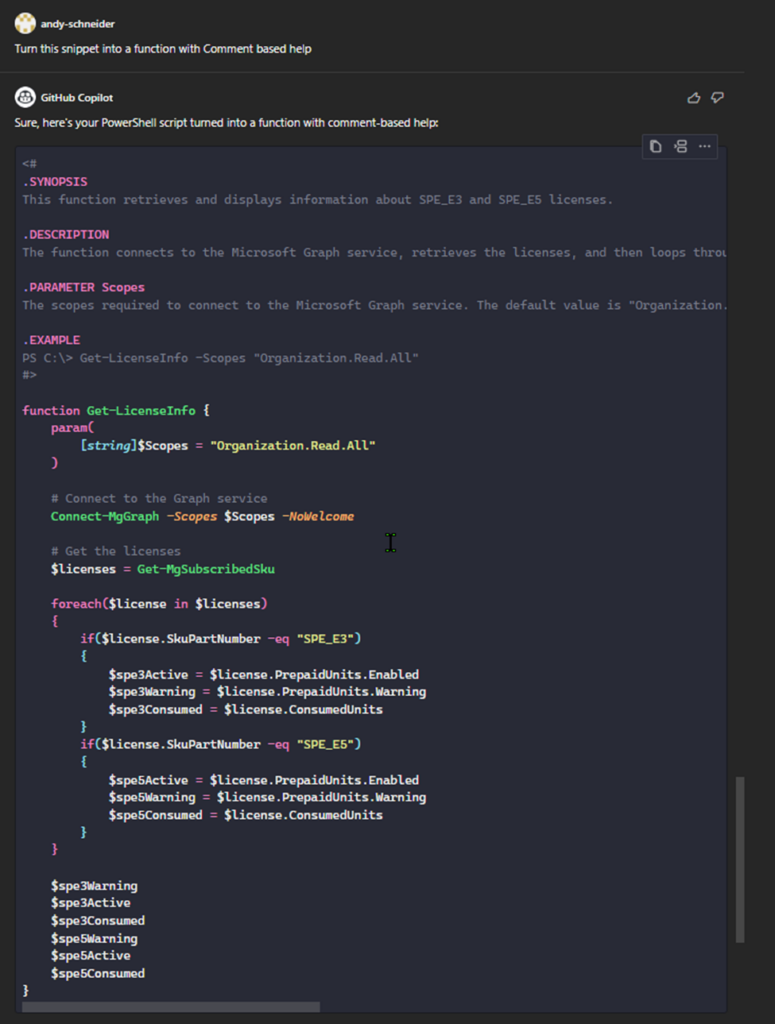
I don’t want to the scope to be a parameter, so I ask Copilot Chat to remove it (Figure 5).
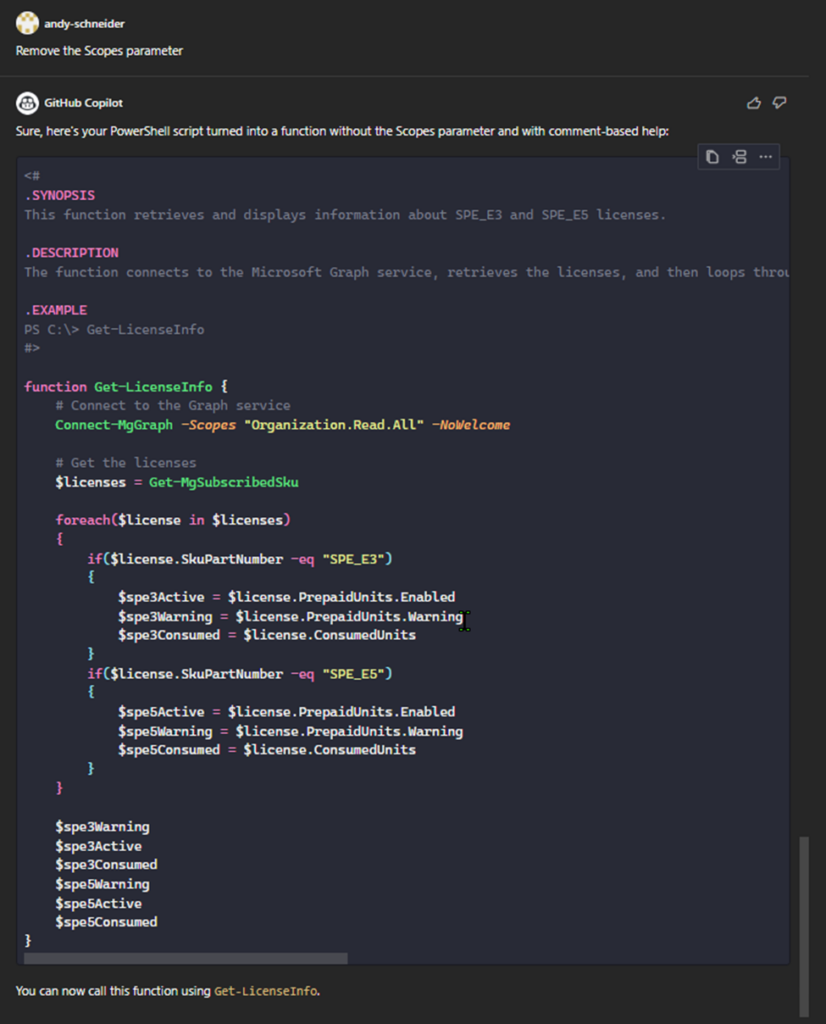
GitHub Copilot does so much menial work for me when I write code that I find myself frustrated when working in an IDE that doesn’t have access to GitHub Copilot.
Is GitHub Copilot perfect? Absolutely not. Everything it produces should be tested and verified. Developing test cases and implementing them should always be part of script development.
Although subtle, I can tell it has changed the way I write code, for me it is absolutely worth $10 a month. If you write PowerShell code on at least a weekly basis, I would say that GitHub Copilot is a worthwhile investment. If you are not quite there yet, give it another shot in 3 to 6 months, and you will likely be pleasantly surprised.
Cybersecurity Risk Management for Active Directory
Discover how to prevent and recover from AD attacks through these Cybersecurity Risk Management Solutions.